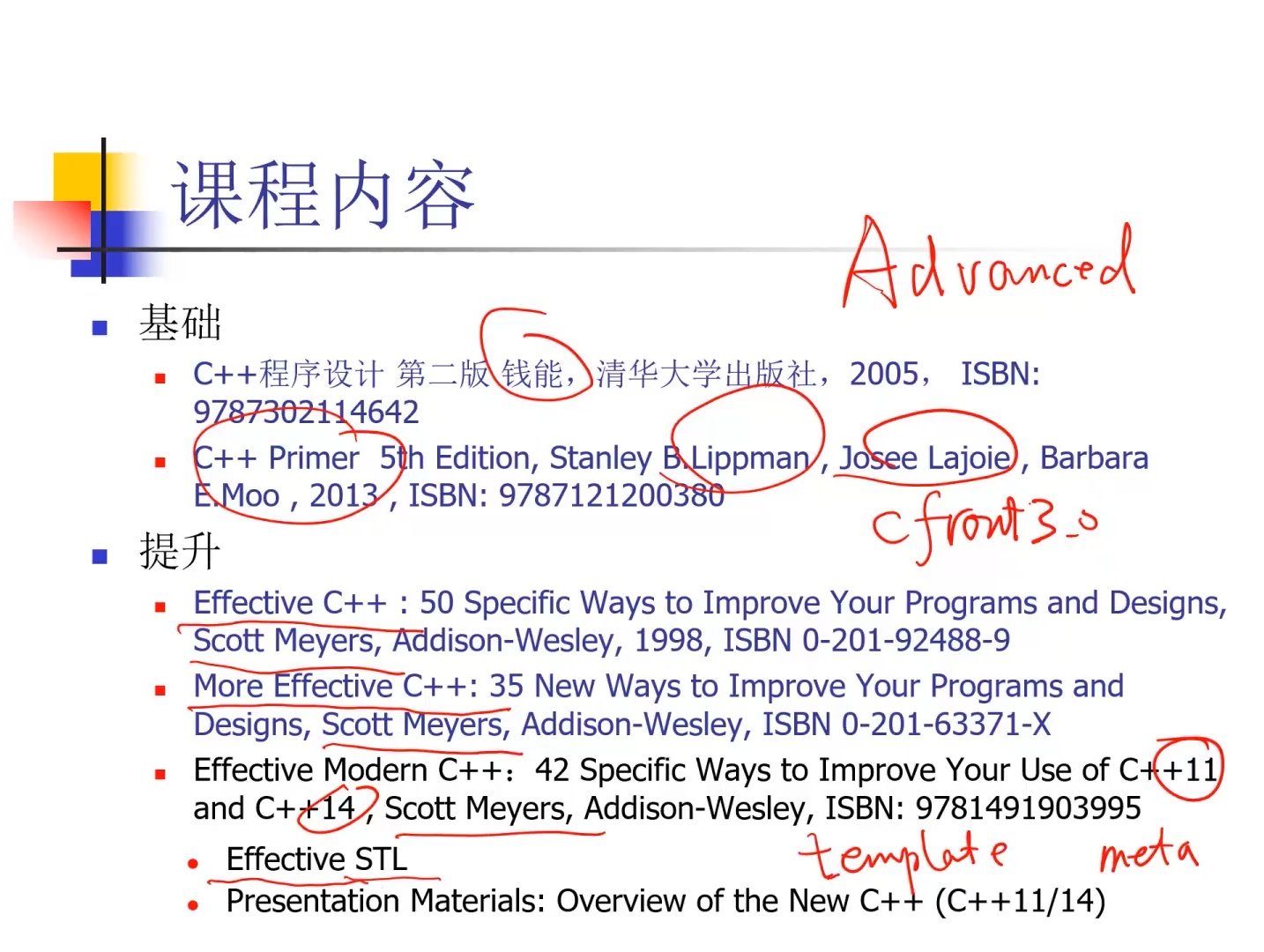
1. 结构化程序设计部分
1.1 数据类型
int x=8;
x的取值范围被称为值集
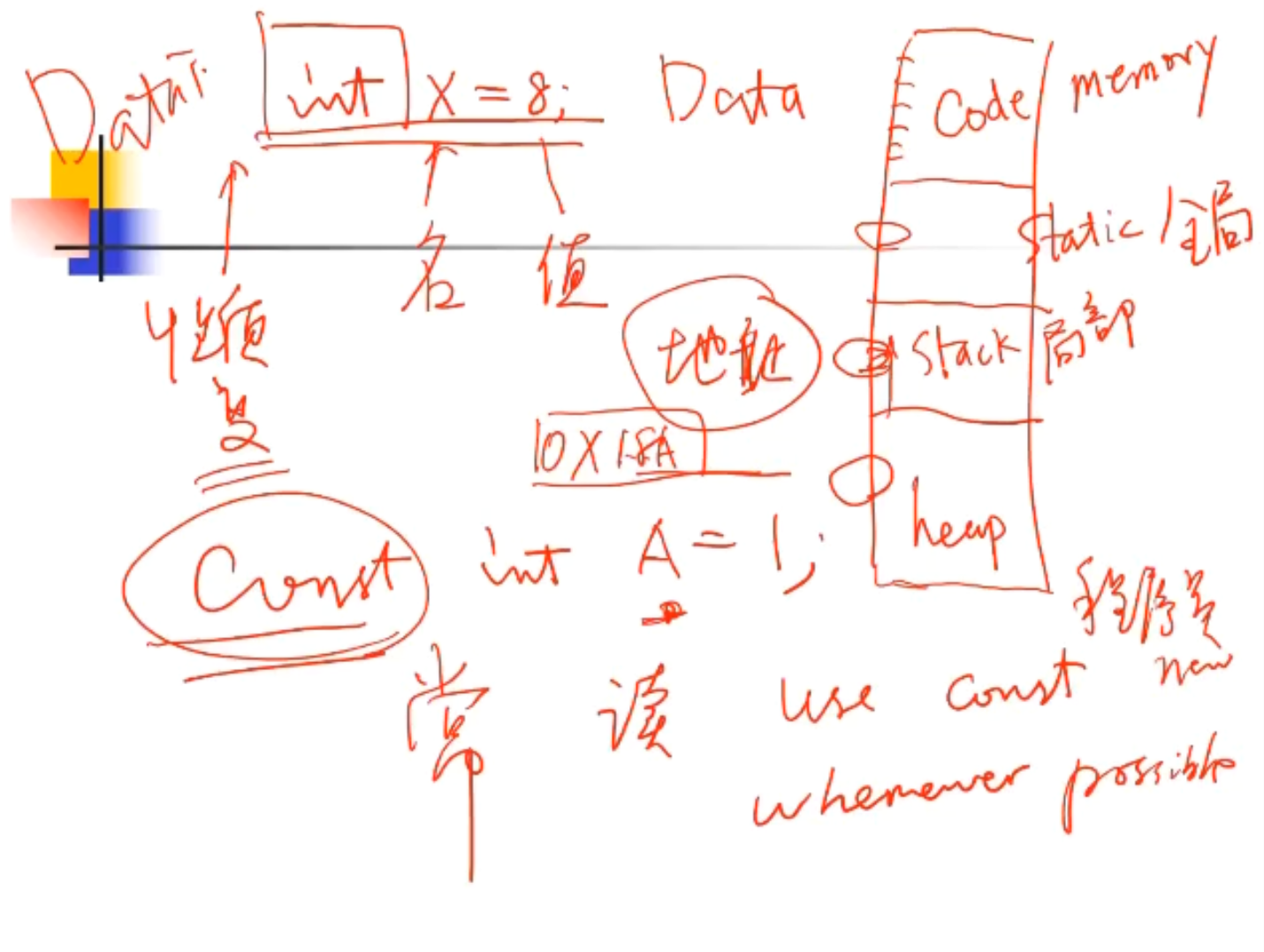
2. 作业部分
2.1 判断int是否溢出
2.1.1 用INT_MAX
INT_MAX这个宏定义了int的最大值,用long long来定义结果变量,如果得到的结果大于INT_MAX则发生了溢出,不能用int定义结果变量,因为是int类型,就算发生了溢出也会截断一些位来使变量保持在int范围内(溢出会发生未定义的行为),所以int类型的变量永远不可能超过INT_MAX。
2.1.2 用数学方法
1 2 3 4 5
| void test(){ int a = 964632435; a = a * 10; cout<<a<<endl; }
|
我们不能通过这样的简单的判断逻辑来进行,发生了溢出,数据已经改变了,不能用改变后的数据(改变后的数据一定是通过了一些丢位操作,使得数值在Int类型的范围内,其实这是未定义的行为)去判断该数值是否溢出。
思想:相加的结果与其中一个数相减,与另一个数比较看是否相等;相乘除的结果和乘除的因子相反操作,和原来的数值进行比较看是否相等。
2.2 最小公倍数和最大公约数
两个数的乘积等于这两个数的最大公约数与最小公倍数的积,可以用欧几里得算法求出最大公约数进而得出最小公倍数。
1 2 3 4 5 6
| int gcd(int a, int b){ if (a%b == 0) { return b; } return gcd(b, a%b); }
|
1 2 3 4 5 6 7 8 9
| int gcd(int a, int b){ int temp = a; while(a%b != 0){ a = b; b = temp%b; temp = a; } return b; }
|
2.3 C++的类
2.3.1 拷贝控制

3. 机考复习
3.1 输入
split函数的实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| vector<string> split(string str, char delimiter) { vector<string> strVec; for (int i = 0; i < str.size(); ++i) { if (str[i] != delimiter) { int j; for (j = i; j < str.size() && str[j] != delimiter; ++j) {
} string temp=str.substr(i,j-i); strVec.push_back(temp); i=j; } } return strVec; }
|
string 头文件中有stoi、stod、stof等函数,可以方便的将字符串转为数字类型。
3.2 STL的使用
std::priority_queue 优先队列的使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115
| #include <iostream> #include <queue> using namespace std;
class Node1 { public: int x; Node1(int x) { this->x = x; } bool operator<(const Node1 &b) const { return x < b.x; } };
class Node2 { public: int x; Node2(int x) { this->x = x; } };
bool operator<(const Node2 &a, const Node2 &b) { return a.x < b.x; }
class Node3 { public: int x; Node3(int x) { this->x = x; } };
class cmpClass { public: bool operator()(const Node3 &a, const Node3 &b) { return a.x < b.x; } };
int main() { priority_queue<Node1> p;
p.emplace(1); p.emplace(3); p.emplace(2);
while (!p.empty()) { cout << p.top().x << " "; p.pop(); } cout << endl;
priority_queue<Node2, vector<Node2>, less<Node2>> q;
q.emplace(1); q.emplace(3); q.emplace(2);
while (!q.empty()) { cout << q.top().x << " "; q.pop(); } cout << endl;
priority_queue<Node3, vector<Node3>, cmpClass> r;
r.emplace(1); r.emplace(3); r.emplace(2);
while (!r.empty()) { cout << r.top().x << " "; r.pop(); } cout << endl; return 0; }
|


3.3 sort算法的使用
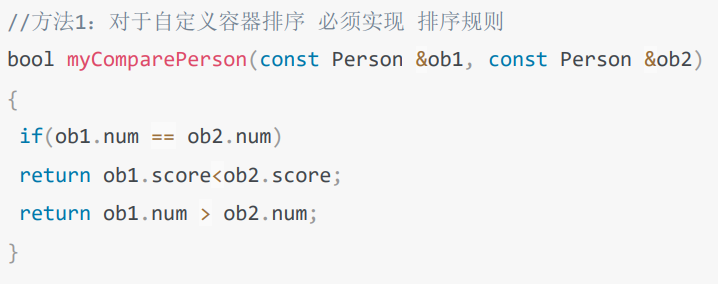
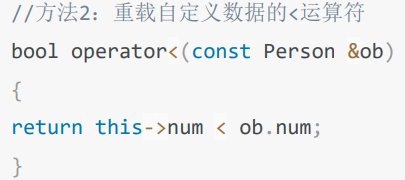
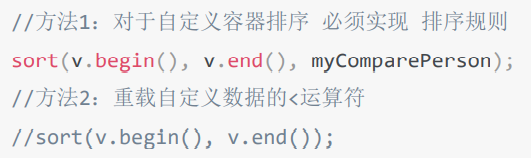
4. 面向对象部分

